ユーザーに広告を表示するためAdMob モジュールを使用します。すべての広告は Google AdMob ネットワーク経由で配信されるため、Google AdMob アカウントが必要になります。
開発環境(Expo Bare)
- “typescript”: “^5.2.2”
- “expo”: “~49.0.13”,
- “react”: “18.2.0”,
- “react-native”: “0.72.6”,
- “expo-router”: “^2.0.0”,
- “react-native-paper”: “^5.10.6”,
- “react-native-google-mobile-ads”: “^12.3.0”,
セットアップ
React Native Google Mobile Ads
パッケージをインストールする
$ yarn add react-native-google-mobile-ads
iOS static frameworksを設定する
// ios/Podfile を編集する ... target 'myproject' do use_expo_modules! config = use_native_modules! // <--- ⬇︎を追加 ---> // use_frameworks! :linkage => :static $RNFirebaseAsStaticFramework = true $RNGoogleMobileAdsAsStaticFramework = true // <--- ⬆︎を追加 ---> // ...
Google AdMob
自身の Google AdMob account でログインしてiOS/Androidアプリを追加する
🥕 Google AdMob > アプリ > アプリを追加
🥕 Google AdMob > iOS or Android アプリを選択 > アプリの設定 > アプリの情報
からアプリ ID
を確認し、app.json
フィアルに追加します。
// <project-root>/app.json { "react-native-google-mobile-ads": { "android_app_id": "ca-app-pub-xxxxxxxx~xxxxxxxx", "ios_app_id": "ca-app-pub-xxxxxxxx~xxxxxxxx" } }
プロジェクトをリビルドする
# For iOS npx pod-install npx react-native run-ios # For Android npx react-native run-android
Outbound requestsを設定する
// app/_layout.tsx import mobileAds, { MaxAdContentRating } from 'react-native-google-mobile-ads'; export default function RootLayout() { const [requestedAds, setRequestedAds] = useState<boolean>(false); ... useEffect(() => { mobileAds() .setRequestConfiguration({ maxAdContentRating: MaxAdContentRating.PG, tagForChildDirectedTreatment: true, tagForUnderAgeOfConsent: true, testDeviceIdentifiers: ['EMULATOR'], }) .then(() => { console.log('🚀AdMob: Request config successfully set!:'); setRequestedAds(true); }); }, []);
Google Mobile Ads SDKを初期化する
// app/_layout.tsx 。。。 useEffect(() => { if (requestedAds) { mobileAds() .initialize() .then(_adapterStatuses => { console.log('🚀AdMob: Initialization complete!:'); }); } }, [requestedAds]); 。。。
App Tracking Transparency (iOS)を設定する
Apple は、IDFA にアクセスするための App Tracking Transparency 認証リクエストを表示することをアプリに要求しています (パーソナライズされた広告を使用するか、パーソナライズされていない広告を使用するかはユーザーに選択を委ねます)。 プロジェクトの app.json または app.config.js ファイル内で、 user_tracking_usage_description を使用して使用方法を説明する必要があります。
{ "react-native-google-mobile-ads": { "android_app_id": "ca-app-pub-xxxxxxxx~xxxxxxxx", "ios_app_id": "ca-app-pub-xxxxxxxx~xxxxxxxx", "user_tracking_usage_description": "This identifier will be used to deliver personalized ads to you." } }
// app/_layout.tsx 。。。 useEffect(() => { (async () => { const {status} = await requestTrackingPermissionsAsync(); if (status === PermissionStatus.GRANTED) { console.log( '🚀AdMob: User permission to track data is', PermissionStatus.GRANTED, ); } })(); }, []); 。。。
※ 詳細
SKAdNetworkを活性化する(iOS)
Google Mobile Ads SDK は、Apple の SKAdNetwork を使用したコンバージョン トラッキングをサポートしています。これにより、Google および参加するサードパーティ購入者は、IDFA が利用できない場合でもアプリのインストールを関連付けることができます。 プロジェクトの app.json
ファイル内に、推奨される SKAdNetwork 識別子 を追加します。
// <project-root>/app.json { "react-native-google-mobile-ads": { "android_app_id": "ca-app-pub-xxxxxxxx~xxxxxxxx", "ios_app_id": "ca-app-pub-xxxxxxxx~xxxxxxxx", "sk_ad_network_items": [ "cstr6suwn9.skadnetwork", "4fzdc2evr5.skadnetwork", "4pfyvq9l8r.skadnetwork", "2fnua5tdw4.skadnetwork", "ydx93a7ass.skadnetwork", "5a6flpkh64.skadnetwork", "p78axxw29g.skadnetwork", "v72qych5uu.skadnetwork", "ludvb6z3bs.skadnetwork", "cp8zw746q7.skadnetwork", "3sh42y64q3.skadnetwork", "c6k4g5qg8m.skadnetwork", "s39g8k73mm.skadnetwork", "3qy4746246.skadnetwork", "f38h382jlk.skadnetwork", "hs6bdukanm.skadnetwork", "v4nxqhlyqp.skadnetwork", "wzmmz9fp6w.skadnetwork", "yclnxrl5pm.skadnetwork", "t38b2kh725.skadnetwork", "7ug5zh24hu.skadnetwork", "gta9lk7p23.skadnetwork", "vutu7akeur.skadnetwork", "y5ghdn5j9k.skadnetwork", "n6fk4nfna4.skadnetwork", "v9wttpbfk9.skadnetwork", "n38lu8286q.skadnetwork", "47vhws6wlr.skadnetwork", "kbd757ywx3.skadnetwork", "9t245vhmpl.skadnetwork", "eh6m2bh4zr.skadnetwork", "a2p9lx4jpn.skadnetwork", "22mmun2rn5.skadnetwork", "4468km3ulz.skadnetwork", "2u9pt9hc89.skadnetwork", "8s468mfl3y.skadnetwork", "klf5c3l5u5.skadnetwork", "ppxm28t8ap.skadnetwork", "ecpz2srf59.skadnetwork", "uw77j35x4d.skadnetwork", "pwa73g5rt2.skadnetwork", "mlmmfzh3r3.skadnetwork", "578prtvx9j.skadnetwork", "4dzt52r2t5.skadnetwork", "e5fvkxwrpn.skadnetwork", "8c4e2ghe7u.skadnetwork", "zq492l623r.skadnetwork", "3rd42ekr43.skadnetwork", "3qcr597p9d.skadnetwork" ] } }
広告を表示する
バナー広告は、既存のアプリケーション内に統合できる部分的な広告です。 インタースティシャル広告やリワード広告とは異なり、バナーはアプリケーションの限られた領域のみを占有し、その領域内に広告を表示します。 これにより、中断を伴う操作を行わずに広告を統合できます。
このモジュールは BannerAd コンポーネントを公開します。 バナーを表示するには、unitId と size プロパティが必要です。
🥕 adUnitId
は Google AdMob > iOS or Androidアプリを選択 > 広告ユニット > 広告ユニットを追加
で追加したバナー広告ユニットの 広告ユニット ID
です。
// app/(tabs)/index.tsx import React from 'react'; import { BannerAd, BannerAdSize, TestIds } from 'react-native-google-mobile-ads'; const adUnitId = __DEV__ ? TestIds.ADAPTIVE_BANNER : 'ca-app-pub-xxxxxxxxxxxxx/yyyyyyyyyyyyyy'; function App() { return ( <BannerAd unitId={adUnitId} size={BannerAdSize.ANCHORED_ADAPTIVE_BANNER} /> ); }
バナー広告の実装ガイドラインを守る
🔥 バナー広告の実装ガイドライン(英語版)の各項目ごとに Click to view an example
テキストをクリックすると推奨方法を図で確認できます!
- ① インタラクティブ要素から広告を分離する!
- ② 広告はアプリのコンテンツから境界線で区切られるように!
- ③ バナー広告に固定スペースを割り当てる!
- ④ 複数のデバイスの画面サイズに対応するアダプティブ バナーを実装する!
Android (Tabbar/No-Tabbar): ①と③を適用!
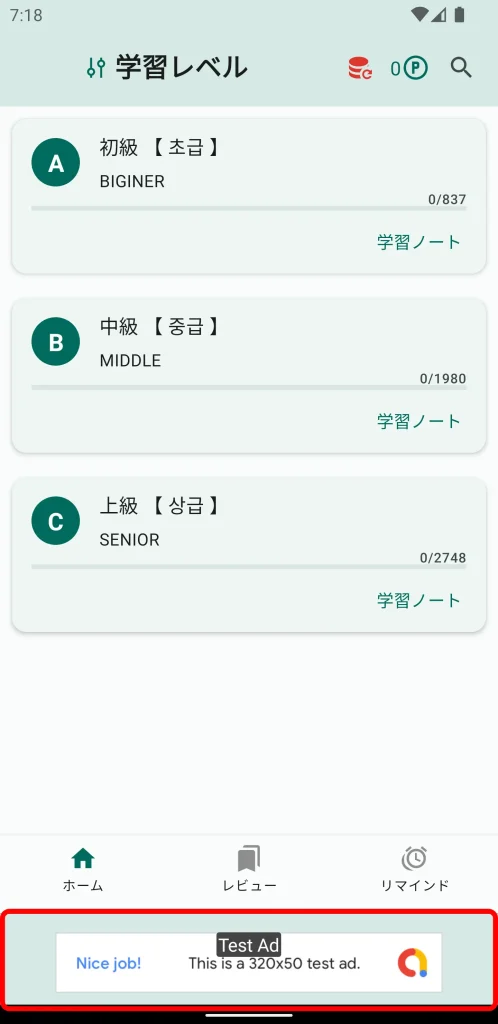
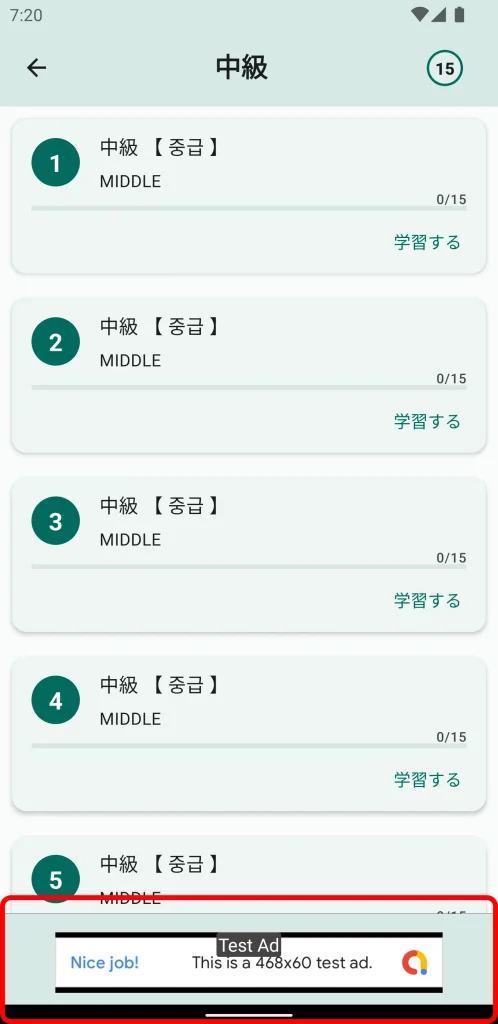
iOS (Tabbar/No-Tabbar): ①と③を適用!
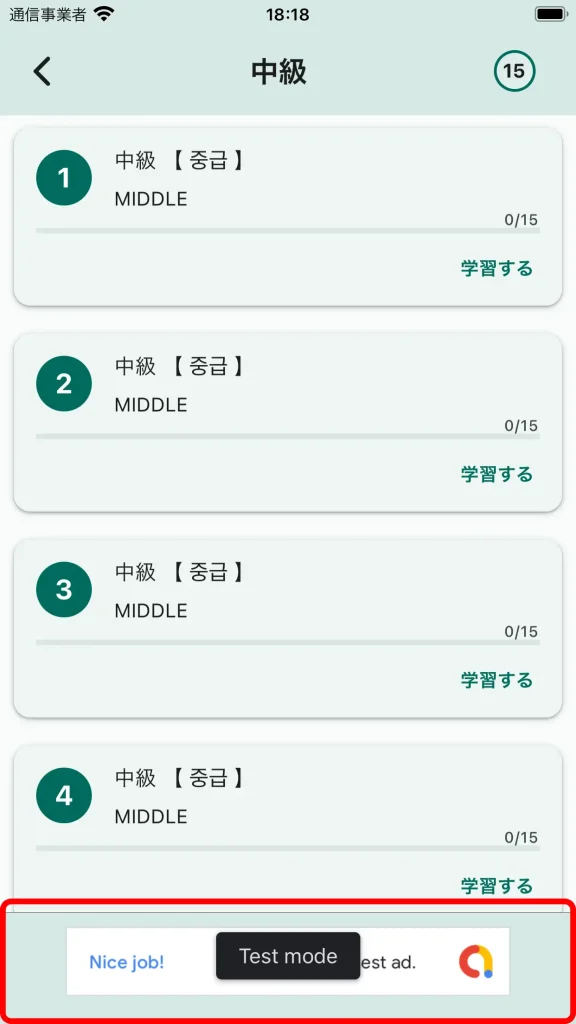
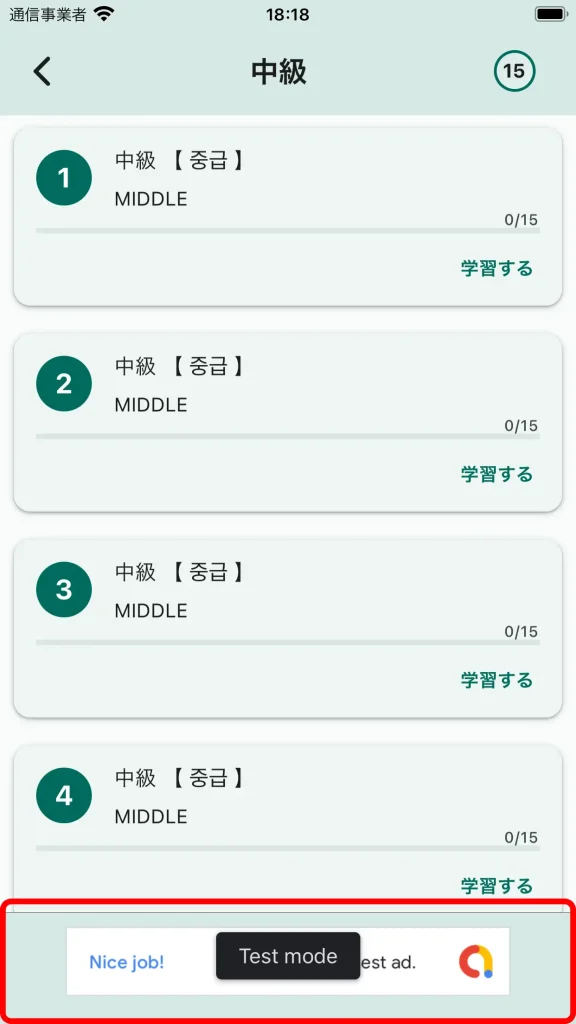
リリースする
※上記の詳細は以下のアプリに実装されています。
コメント